以下では、InputManCellについて解説しますが、PlusPakCellも基本的には同じ機能を提供します。
![]() |
この機能はタッチ操作だけでなく、マウスやキーボードによる操作でも利用できます。 |
セルのスピン機能
一部のInputManCellとPlusPakCellで使用可能なスピン機能は、サイドボタンにSpinButton(スピンボタン)を追加することで実現可能ですが、スピンボタンはマウスによる操作を前提としたボタンの大きさや配置となっており、タッチ操作には適していません。

(図)スピンボタン
InputManCellとPlusPakCellでは、SideButton(サイドボタン)やSymbolButton(シンボルボタン)を使用して、タッチ操作しやすいボタンをカスタマイズする機能を提供します。サイドボタンやシンボルボタンを使用すると、タッチしやすいボタンの大きさを提供でき、またボタンを水平に隣接したり、セルの両端に配置することも可能です。
これらのボタンにスピン機能を設定する手順は以下のとおりです。

(図)スピン機能を割り当てたサイドボタンの例

(図)スピン機能を割り当てたシンボルボタンの例
各セルのスピン機能やサイドボタンの詳細については、それぞれ下記のページを参照してください。

(図)スピンボタン
InputManCellとPlusPakCellでは、SideButton(サイドボタン)やSymbolButton(シンボルボタン)を使用して、タッチ操作しやすいボタンをカスタマイズする機能を提供します。サイドボタンやシンボルボタンを使用すると、タッチしやすいボタンの大きさを提供でき、またボタンを水平に隣接したり、セルの両端に配置することも可能です。
これらのボタンにスピン機能を設定する手順は以下のとおりです。
- セルにサイドボタンあるいはシンボルボタンを2つ追加します。
- 各ボタンにスピン機能を割り当てます。一つのボタンにはスピンアップ機能を、もう一つにはスピンダウン機能を設定します。
- 必要に応じて、Positionプロパティによりボタンの配置を設定します。
- 必要に応じて、ボタンに表示するテキストやシンボル、あるいは画像などを設定します。

(図)スピン機能を割り当てたサイドボタンの例

(図)スピン機能を割り当てたシンボルボタンの例
各セルのスピン機能やサイドボタンの詳細については、それぞれ下記のページを参照してください。
- InputManCell
- GcMaskCell−サイドボタンを設定する
- GcDateTimeCell−サイドボタンを設定する
- GcTimeSpanCell−サイドボタンを設定する
- GcNumberCell−サイドボタンを設定する
- GcComboBoxCell−サイドボタンを設定する
- PlusPakCell
- GcFontPickerCell−セルのカスタマイズ
サイドボタンの利用
ボタンに「+」「−」といった任意のテキストや画像を設定したい場合は、SideButton(サイドボタン)を使用します。サイドボタンにスピン機能を割り当てるには、Behaviorプロパティを使用します。また、Behaviorプロパティに設定した値により、IntervalプロパティとTextプロパティの初期値が自動的に変更されます。
Behaviorプロパティに設定可能な値は以下の通りです。
以下のサンプルコードは、GcNumberCellにスピン機能を割り当てたサイドボタンを追加する例です。セルの左端にスピンダウン機能を持つボタン、右端にスピンアップ機能を持つボタンを設定します。

(図)上記サンプルコードの実行結果
Behaviorプロパティに設定可能な値は以下の通りです。
Behaviorの値 | 説明 | Intervalプロパティの初期値 | Textプロパティの初期値 |
---|---|---|---|
None | スピン動作を割り当てません。 | 0 | なし |
SpinUp | スピンアップ(値を増加)の動作を割り当てます。 | 60 | "+" |
SpinDown | スピンダウン(値を減少)の動作を割り当てます。 | 60 | "-" |
以下のサンプルコードは、GcNumberCellにスピン機能を割り当てたサイドボタンを追加する例です。セルの左端にスピンダウン機能を持つボタン、右端にスピンアップ機能を持つボタンを設定します。
Imports GrapeCity.Win.MultiRow Imports InputManCell = GrapeCity.Win.MultiRow.InputMan Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load Dim GcNumberCell1 = New InputManCell.GcNumberCell() GcNumberCell1.Name = "GcNumberCell1" GcNumberCell1.Size = New Size(120, 20) ' セルのサイドボタンをクリアします。 GcNumberCell1.SideButtons.Clear() ' スピンダウン機能を持つサイドボタンを設定します。 Dim DownButton = New InputManCell.SideButton() DownButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinDown DownButton.Position = LeftRightAlignment.Left ' セルにサイドボタンを追加します。 GcNumberCell1.SideButtons.Add(DownButton) ' スピンアップ機能を持つサイドボタンを設定します。 Dim UpButton = New InputManCell.SideButton() UpButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinUp ' セルにサイドボタンを追加します。 GcNumberCell1.SideButtons.Add(UpButton) GcMultiRow1.Template = Template.CreateGridTemplate(New Cell() {GcNumberCell1}) GcMultiRow1.RowCount = 5 End Sub
using GrapeCity.Win.MultiRow; using InputManCell = GrapeCity.Win.MultiRow.InputMan; private void Form1_Load(object sender, EventArgs e) { InputManCell.GcNumberCell gcNumberCell1 = new InputManCell.GcNumberCell(); gcNumberCell1.Name = "gcNumberCell1"; gcNumberCell1.Size = new Size(120, 20); // セルのサイドボタンをクリアします。 gcNumberCell1.SideButtons.Clear(); // スピンダウン機能を持つサイドボタンを設定します。 InputManCell.SideButton DownButton = new InputManCell.SideButton(); DownButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinDown; DownButton.Position = LeftRightAlignment.Left; // セルにサイドボタンを追加します。 gcNumberCell1.SideButtons.Add(DownButton); // スピンアップ機能を持つサイドボタンを設定します。 InputManCell.SideButton UpButton = new InputManCell.SideButton(); UpButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinUp; // セルにサイドボタンを追加します。 gcNumberCell1.SideButtons.Add(UpButton); gcMultiRow1.Template = Template.CreateGridTemplate(new Cell[] { gcNumberCell1 }); gcMultiRow1.RowCount = 5; }

(図)上記サンプルコードの実行結果
スピンボタンをシンボルボタンへ置き換え
ボタンに提供されたイメージを設定したい場合は、SymbolButton(シンボルボタン)を使用します。シンボルボタンにスピン機能を割り当てるには、Behaviorプロパティを使用します。また、Behaviorプロパティに設定した値により、Intervalプロパティ、SymbolプロパティおよびSymbolDirectionプロパティの初期値が自動的に変更されます。
Behaviorプロパティに設定可能な値は以下の通りです。
以下のサンプルコードは、日付コントロールにスピン機能を割り当てたシンボルボタンで実装する例です。コントロールの左端にスピンダウン機能を持つボタン、右端にスピンアップ機能を持つボタンを設定します。
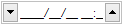
(図)上記サンプルコードの実行結果
Behaviorプロパティに設定可能な値は以下の通りです。
Behaviorの値 | 説明 | Intervalプロパティの初期値 | Symbolプロパティの初期値 | SymbolDirectionプロパティの初期値 |
---|---|---|---|---|
None | スピン動作を割り当てません。 | 0 | None | Left |
SpinUp | スピンアップ(値を増加)の動作を割り当てます。 | 60 | Arrow | Up |
SpinDown | スピンダウン(値を減少)の動作を割り当てます。 | 60 | Arrow | Down |
以下のサンプルコードは、日付コントロールにスピン機能を割り当てたシンボルボタンで実装する例です。コントロールの左端にスピンダウン機能を持つボタン、右端にスピンアップ機能を持つボタンを設定します。
Imports GrapeCity.Win.MultiRow Imports InputManCell = GrapeCity.Win.MultiRow.InputMan Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load Dim GcDateTimeCell1 = New InputManCell.GcDateTimeCell() GcDateTimeCell1.Name = "GcDateTimeCell1" GcDateTimeCell1.Size = New Size(120, 20) ' セルのサイドボタンをクリアします。 GcDateTimeCell1.SideButtons.Clear() ' スピンダウン機能を持つシンボルボタンを設定します。 Dim DownButton As New InputManCell.SymbolButton() DownButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinDown DownButton.Position = LeftRightAlignment.Left ' セルにシンボルボタンを追加します。 GcDateTimeCell1.SideButtons.Add(DownButton) ' スピンアップ機能を持つシンボルボタンを設定します。 Dim UpButton As New InputManCell.SymbolButton() UpButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinUp ' セルにシンボルボタンを追加します。 GcDateTimeCell1.SideButtons.Add(UpButton) GcMultiRow1.Template = Template.CreateGridTemplate(New Cell() {GcDateTimeCell1}) GcMultiRow1.RowCount = 5 End Sub
using GrapeCity.Win.MultiRow; using InputManCell = GrapeCity.Win.MultiRow.InputMan; private void Form1_Load(object sender, EventArgs e) { InputManCell.GcDateTimeCell gcDateTimeCell1 = new InputManCell.GcDateTimeCell(); gcDateTimeCell1.Name = "gcDateTimeCell1"; gcDateTimeCell1.Size = new Size(120, 20); // セルのサイドボタンをクリアします。 gcDateTimeCell1.SideButtons.Clear(); // スピンダウン機能を持つシンボルボタンを設定します。 InputManCell.SymbolButton DownButton = new InputManCell.SymbolButton(); DownButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinDown; DownButton.Position = LeftRightAlignment.Left; // セルにシンボルボタンを追加します。 gcDateTimeCell1.SideButtons.Add(DownButton); // スピンアップ機能を持つシンボルボタンを設定します。 InputManCell.SymbolButton UpButton = new InputManCell.SymbolButton(); UpButton.Behavior = GrapeCity.Win.Editors.SideButtonBehavior.SpinUp; // セルにシンボルボタンを追加します。 gcDateTimeCell1.SideButtons.Add(UpButton); gcMultiRow1.Template = Template.CreateGridTemplate(new Cell[] { gcDateTimeCell1 }); gcMultiRow1.RowCount = 5; }
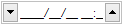
(図)上記サンプルコードの実行結果